
Hello, and welcome to DiscordCoreAPI! This is a Discord bot library, written in C++, that leverages custom asynchronous CoRoutines, as well as a home-brew set of Https, WebSocket, and Datagram socket clients - all to deliver the utmost performance and efficiency for your bot. It uses roughly 0.1% of an Intel i7 9750h CPU to stream audio in high quality (Opus @ 48Khz, 16-bit) to a single server.
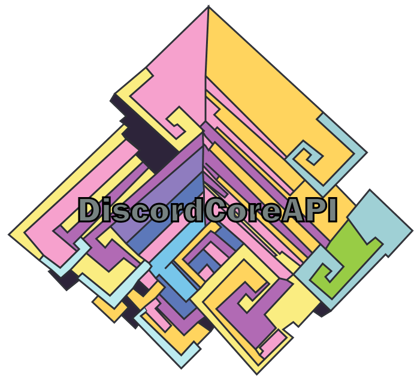
Compiler Support

Operating System Support

Documentation/Examples
C++ Discord Bot Documentation/Examples
Discord Server
This is a link to the Discord server!
Bot Template
A template for utilizing this library.
Features
Convert Snowflakes Into Data
- Using the
discord_core_api::snowflake::toEntity()
function of the discord_core_api::snowflake
class allows for converting the entity's id directly into the data structure represented by it.
std::cout <<
"CHANNEL NAME: " + newChannel.
name << std::endl;
jsonifier::string name
The name of the channel (1-100 characters).
A class representing a snowflake identifier with various operations.
Performant
Audio-Bridge
CPU Efficient
- It only uses about 0.1% of an Intel i7 9750h to stream audio in high quality (Opus 48Khz 16-bit Stereo) to a single server.
Entire Discord API Covered
- All of the Discord API endpoints are covered in this library, including voice communication.
Concurrent Discord API Access
Advanced Rate-Limiting System
- Guarantees that the order in which HTTPS requests are executed is the same that they were submitted in - despite being launched across different threads, while never infracting on any of the Discord API's rate-limits and while running concurrently across all of the endpoints.
Slash Commands and Buttons
Select Menus
User Commands
Message Commands
Modal Text Inputs
A Unified "Input-Event" System
- User interactions (Application Commands, Message Commands, User Commands) are accepted via the
discord_core_api::event_manager::onInputEventCreation
event.
- They can all be responded to using the
discord_core_api::input_events::respondToInputEventAsync()
function.
- Alternatively you can implement your own input-event handling by using the raw
discord_core_api::event_manager::onInteractionCreation
or discord_core_api::event_manager::onMessageCreation
events. EmbedData newEmbed{};
newEmbed.setAuthor(args.eventData.getUserName(), args.eventData.getAvatarURL());
newEmbed.setDescription("------\\n__**Sorry, but there's already something playing!**__\\n------");
newEmbed.setTimeStamp(getTimeAndDate());
newEmbed.setTitle("__**Playing Issue:**__");
newEmbed.setColor(discordGuild.data.borderColor);
RespondToInputEventData dataPackage{ args.eventData };
dataPackage.addMessageEmbed(newEmbed);
dataPackage.setResponseType(InputEventResponseType::Ephemeral_Interaction_Response);
InputEventData newEvent = input_events::respondToInputEvent(dataPackage);
input_events::deleteInputEventResponseAsync(newEvent, 20000).get();
Build Instructions (Full-Vcpkg)
- Install vcpkg, if need be.
- Make sure to run
vcpkg integrate install
.
- Enter within a terminal
vcpkg install discordcoreapi:x64-windows_OR_linux
.
- Set up a console project in your IDE and make sure to set the C++ standard to C++20 or later - and include
discord_core_api::discordcoreapi/Index.hpp
.
- Build and run!
Dependencies
- CMake (Version 3.20 or greater)
- NOTE: I installed these using the vcpkg installer.
- Jsonifier (.\vcpkg install jsonifier:x64-windows_OR_linux)
- OpenSSL (.\vcpkg install openssl:x64-windows_OR_linux)
- Opus (.\vcpkg install opus:x64-windows_OR_linux)
- Sodium (.\vcpkg install libsodium:x64-windows_OR_linux)
Build Instructions (Non-Vcpkg) - The Library
- Install the dependencies.
- Clone this git repository into a folder.
- Set, in CMakeLists.txt, the
_VCPKG_ROOT_DIR
, or the Opus_DIR
, unofficial-sodium_DIR
paths to wherever each of the respective dependency files are located and they are as follows:
- Opus_DIR # Set this one to the folder location of the file "OpusConfig.cmake".
- unofficial-sodium_DIR # Set this one to the folder location of the file "unofficial-sodiumConfig.cmake".
- OPENSSL_ROOT_DIR # Set this one to the folder location of the include folder and library folders of OpenSSL.
- Open a terminal inside the git repo's folder.
- Run
cmake -S . --preset Linux_OR_Windows-Debug_OR_Release
.
- Then run
cmake --build --preset Linux_OR_Windows-Debug_OR_Release
.
- Run within the same terminal and folder
cmake --install ./Build/Debug_OR_Release
.
- The default installation paths are: Windows = "ROOT_DRIVE:/Users/USERNAME/CMake/DiscordCoreAPI", Linux = "/home/USERNAME/CMake/DiscordCoreAPI"
The CMAKE Package
- By running
cmake --install ./Build/Debug_OR_Release
, you will be given a cmake package, which can be used to build from this library, using other cmake projects.
- It is used by setting
DiscordCoreAPI_DIR
to wherever the DiscordCoreAPIConfig.cmake file would have been installed on your system by having run the cmake --install
command, and then using find_package()
on DiscordCoreAPI
.
- When found, you will be granted the following cmake "variables";
DiscordCoreAPI::DiscordCoreAPI
- this is the library target which can be linked to from other targets in cmake, and on Windows; $<TARGET_RUNTIME_DLLS:DiscordCoreAPI-Bot>
- which is a list of dll files to be copied into your executable's final location after building. As well as RELEASE_PDB_FILE_PATH
, DEBUG_PDB_FILE_PATH
, RELEASE_PDB_FILE_NAME
, and DEBUG_PDB_FILE_NAME
, which are full file/directory paths/filenames to the library's PDB files.
- Here is an example of building an executable from this library with this method.
Build Instructions (Non-Vcpkg) - The Executable
- Download the bot template or create your own with the same imports, and set within it either the
VCPKG_ROOT_DIR
, or the CMAKE_CONFIG_FILE_DIR
, Opus_DIR
, and unofficial-sodium_DIR
paths to wherever each of the respective dependency files are located and they are as follows:
- CMAKE_CONFIG_FILE_DIR # Set this one to the folder location of the DiscordCoreAPIConfig.cmake generated while running CMake –install.
- Opus_DIR # Set this one to the folder location of the file "OpusConfig.cmake".
- unofficial-sodium_DIR # Set this one to the folder location of the file "unofficial-sodiumConfig.cmake".
- OPENSSL_ROOT_DIR # Set this one to the folder location of the include folder and library folders of OpenSSL.
- Set up a main.cpp like this one, including the header
discordcoreapi/Index.hpp
.
- Run in a terminal from within the same folder as the top-level CMakeLists.txt,
cmake -S . --preset Linux_OR_Windows-Debug_OR_Release
.
- Then run
cmake --build --preset Linux_OR_Windows-Debug_OR_Release
.
- Run within the same terminal and folder
cmake --install ./Build/Debug_OR_Release
.
- The default installation paths are: Windows = "ROOT_DRIVE:/Users/USERNAME/CMake/Bot-Template-For-DiscordCoreAPI", Linux = "/home/USERNAME/CMake/Bot-Template-For-DiscordCoreAPI"